react-native-word-wrap-text
Simple word-wrapped text component for React Native.
Features
- Support TypeScript
- Line-break using
n
in text
Next Features
- Remove unnecessary empty
TextRenderer
Requirements
🚨 This module using lookbehind regular expression, you must enable hermes. Hermes makes you using ESnext features.
Enable hermes like below.
// /android/app.build.gradle project.ext.react = [ enableHermes: true, // modify to true ]
and clean gradle by run command in /android
Installation
npm install react-native-word-wrap-text
or
yarn add react-native-word-wrap-text
Usage
import {Text, View} from 'react-native'; import { TextAndRendererList, TextRenderer, WordWrapText, } from 'react-native-word-wrap-text'; const renderPlainText: TextRenderer = (token, index) => { return ( // it's important to assign key props to root component <Text key={index} style={textStyle.plainText}> {token} </Text> ); }; const renderPrimaryText: TextRenderer = (token, index) => { return ( <Text key={index} style={textStyle.primaryText}> {token} </Text> ); }; const renderBoldText: TextRenderer = (token, index) => { return ( <Text key={index} style={textStyle.boldText}> {token} </Text> ); }; // if use same renderer, merge to one string const textAndRendererList: TextAndRendererList = [ ['This library is easily customizable. ', renderPlainText], ['Now, it supports ', renderPrimaryText], ['\n ', renderBoldText], ['for line-break. ', renderPrimaryText], ['thank fornusing this library.', renderPlainText], ]; function SomeComponent (props) { // ... return ( <View> <WordWrapText textAndRenderList={textAndRendererList} containerStyle={containerStyle.text} /> </View> ); }
🚨 You have to assign
key
prop to root component at TextRenderer
Props and Types
Props
Props | type | note |
---|---|---|
textAndRenderList | TextAndRendererList | |
containerStyle | StyleProp<ViewStyle> | View Style Props |
Types
Types | value | note |
---|---|---|
TextRenderer | (token: string, index: number) => ReactNode | Similar to function component |
TextAndRenderer | [string, TextRenderer] | string and TextRenderer tuple |
TextAndRendererList | TextAndRenderer[] | 2D array |
Contributing
See the contributing guide to learn how to contribute to the repository.
License
MIT
Getting the text to wrap because flexWrap ain’t cuttin’ it
I don’t know about you, but React Native’s Layout and Flexbox model is confusing sometimes. That’s because it doesn’t always behave like it does in CSS. Really all you want is React Native text overflow to just wrap and call it a day. But it’s not so straightforward like that.
Lets wrap your head around flexWrap
In React Native, you may be tempted to try the flexWrap because it’s the only option with the word “wrap” in it. There’s even some people recommending using that style, but that style’s main concern is something else: it’s to break flexbox children, not text.
After some research, I found this GitHub issue.
Now, the solutions that were successful used the flexWrap option, but upon testing the flexWrap option was redundant. Some of the solutions used “flex: 1” on the parent view. I personally didn’t want to do that since that would negatively affect the layout of my app. I wanted my particular parent view to grow to the available space that was left.
If you understand “flex: 1” you’ll know that it’ll distribute the dimension in relationship to its siblings.
Lets say you do the following:
How flex works. It’s not related to your muscles.
If you understand “flex: 1” you’ll know that it’ll distribute the dimension in relationship to its siblings.
Lets say you do the following:
import * as React from "react";
import {View} from "react-native";
One
Two
Three
Notice how there’s three children with “flex: 1”. In this case, the default flexbox direction is column (or vertical), so each children’s height will be distributed equally 1 + 2 + 1 = 4. So the first and last child takes 1/4th of the height, while the middle child gets 2/4ths of the height (or 1/2).
Going back to the text wrap issue in React Native. What I wanted to do was only have the text breaking and not affecting the overall flexbox layout. So in order to do that you’ll need to apply the “flex: 1” to the Text component itself, and for the parent you’ll add “flexDirection: row“.
Here’s how that looks like:
import * as React from 'react';
import { Text, View, SafeAreaView } from 'react-native';
export default function App() {
return (
One
Two very long text
Three
);
}
If you run the previous code you’ll see that it works just fine on native devices, but the text doesn’t wrap for web. So to fix that just add “width: 1” to the Text component like so.
Two very long text
The secret overflow sauce
It’s this specific combination of parent and child styles that break texts for both native devices and web. If you don’t want to add the “width: 1” across all platforms, you could just use “Platform.OS” and conditionally check for “web”.
So now lets see how that looks with the fix:
import * as React from 'react';
import { Text, View, SafeAreaView } from 'react-native';
export default function App() {
return (
One
Two very long text
Three
);
}
I’ve tried that in Brave (essentially Chrome), Safari, Firefox, and Microsoft Edge. They all accept the fix. It doesn’t seem like it’s something officially documented as I’ve tried to look up the W3 docs and there’s nothing that would allude to doing this. So consider this a “hack” just like back in the old days when we had to support IE 6+ ().
The only thing that I could assume here is that the flex width of the parent is growing to the full size of its child because of “flexGrow: 1” and then adding “width: 1” makes the flex child shrink less than the threshold causing “flexGrow: 1” to use the remainder of the width available from the parent container’s 160 total width. Basically 160 – 50 – 50 (container width minus the 2 views with 50) left us with 60 and that’s what flexGrow used instead of the intrinsic width of the text context which was longer than 60 units (px in web).
I hope this was useful to you and you’re able to create cross-platform React Native apps.
-
Expo, React Native, React Native word break, wrap content
The following code can be found in this live example
I’ve got the following react native element:
'use strict';
var React = require('react-native');
var {
AppRegistry,
StyleSheet,
Text,
View,
} = React;
var SampleApp = React.createClass({
render: function() {
return (
<View style={styles.container}>
<View style={styles.descriptionContainerVer}>
<View style={styles.descriptionContainerHor}>
<Text style={styles.descriptionText} numberOfLines={5} >
Here is a really long text that you can do nothing about, its gonna be long wether you like it or not, so be prepared for it to go off screen. Right? Right..!
</Text>
</View>
</View>
<View style={styles.descriptionContainerVer2}>
<View style={styles.descriptionContainerHor}>
<Text style={styles.descriptionText} numberOfLines={5} >Some other long text which you can still do nothing about.. Off the screen we go then.</Text>
</View>
</View>
</View>);
}
});
AppRegistry.registerComponent('SampleApp', () => SampleApp);
with the following styles:
var styles = StyleSheet.create({
container:{
flex:1,
flexDirection:'column',
justifyContent: 'flex-start',
backgroundColor: 'grey'
},
descriptionContainerVer:{
flex:0.5, //height (according to its parent)
flexDirection: 'column', //its children will be in a row
alignItems: 'center',
backgroundColor: 'blue',
// alignSelf: 'center',
},
descriptionContainerVer2:{
flex:0.5, //height (according to its parent)
flexDirection: 'column', //its children will be in a row
alignItems: 'center',
backgroundColor: 'orange',
// alignSelf: 'center',
},
descriptionContainerHor:{
//width: 200, //I DON'T want this line here, because I need to support many screen sizes
flex: 0.3, //width (according to its parent)
flexDirection: 'column', //its children will be in a column
alignItems: 'center', //align items according to this parent (like setting self align on each item)
justifyContent: 'center',
flexWrap: 'wrap'
},
descriptionText: {
backgroundColor: 'green',//Colors.transparentColor,
fontSize: 16,
color: 'white',
textAlign: 'center',
flexWrap: 'wrap'
}
});
This results in the following screen:
How can I stop the text from going off the screen and keep it confined in the middle of the screen with a width of i.e. 80% of the parent.
I don’t think I should use width
because I will be running this on MANY different mobile screens and I want it to be dynamic, so I think I should rely totally on flexbox
.
(That was the initial reason why I had flex: 0.8
within the descriptionContainerHor
.
What I want to achieve is something like this:
Thank you!
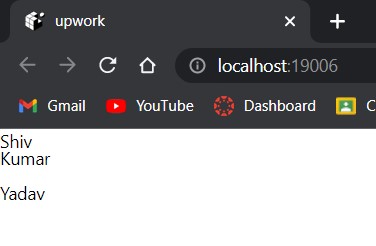
Today’s tutorial demonstrates wrapping text in React Native using different code examples.
Text Wrap in React Native
Since flex-wrap is the only option in React Native containing the word wrap
, you might be inclined to try it.
While some advocate utilizing such a style, its biggest drawback is that it can break flexbox children rather than text, not the text itself.
The practical solutions employed the flex-wrap option, although testing revealed that it was unnecessary. flex: 1
was utilized in a few of the solutions on the parent view.
We didn’t want to do that since it would have affected the design of my software. So instead, we desired my specific parent view to fill the remaining area.
Understanding flex: 1
will allow you to know how the dimension will be distributed among its siblings.
Let’s say you carry out the following:
import { View, Text } from "react-native";
import React from "react";
function App() {
return (
<>
<View>
<View style={{ flex: 1 }}>
<Text>Shiv</Text>
</View>
<View style={{ flex: 2 }}>
<Text>Kumar</Text>
</View>
<View style={{ flex: 1 }}>
<Text>Yadav</Text>
</View>
</View>
</>
);
}
export default App;
Output:
Since the default flexbox orientation in this situation is column (or vertical), the heights of each child will be divided evenly, making 1 + 2 + 1 = 4
.
The middle kid receives 2/4ths
of the height (or 1/2
), while the first and last children each receive 1/4
of the height.
Returning to the React Native text wrap problem. We wanted the text to break; we didn’t want the entire flexbox layout to be affected.
Therefore, you must add flex: 1
to the Text
component and flex-direction: row
to the parent.
This is how it appears:
import { View, Text, SafeAreaView } from "react-native";
import React from "react";
function App() {
return (
<>
<SafeAreaView style={{ flex: 1, flexDirection: "row", width: 160 }}>
<View style={{ width: 50 }}>
<Text>Shiv</Text>
</View>
<View style={{ flexGrow: 1, flexDirection: "row" }}>
<Text style={{ flex: 1 }}>This is how work </Text>
</View>
<View style={{ width: 50 }}>
<Text>Yadav</Text>
</View>
</SafeAreaView>
</>
);
}
export default App;
SafeAreaView
renders the content within a device’s boundary.
Output:
If you execute the code above, you’ll observe that it functions flawlessly on native devices but fails to wrap the text for the web properly. Add width: 1
to the Text
component as shown to correct that.
import { View, Text, SafeAreaView } from "react-native";
import React from "react";
function App() {
return (
<>
<SafeAreaView style={{ flex: 1, flexDirection: "row", width: 160 }}>
<View style={{ width: 50 }}>
<Text>Shiv</Text>
</View>
<View style={{ flexGrow: 1, flexDirection: "row" }}>
<Text style={{ flex: 1, width: 1 }}>This is how work </Text>
</View>
<View style={{ width: 50 }}>
<Text>Yadav</Text>
</View>
</SafeAreaView>
</>
);
}
export default App;
Output:
Aug 03, 2022 . Admin
In this tutorial, you will learn react native text component wrap. We will look at example of react native text wrap to next line. you can see how to wrap text in react native. In this article, we will implement a how to wrap text in react native. Let’s get started with react native text wrap break word.
Sometimes, we need to wrap text in react native on screen. here we will add To wrap React Native text on the screen, we can set flexWrap to ‘wrap’. so let’s see simple example
Step 1: Download Project
In the first step run the following command to create a project.
expo init ExampleApp
Step 2: App.js
In this step, You will open the App.js file and put the code.
import React from "react"; import { StyleSheet, Text, View} from 'react-native'; export default function App() { return ( <View style={{ flexDirection: 'row',alignItems: 'center' ,justifyContent: 'center',flex:1}}> <Text style={{ flex: 1, flexWrap: 'wrap' }}> <View> <Text>Lorem ipsum dolor sit amet, consectetur adipisicing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.</Text> </View> <View> <Text>Lorem ipsum dolor sit amet, consectetur adipiscing elit.</Text> </View> </Text> </View> ); }
Step 3: Run Project
In the last step run your project using the below command.
expo start
You can QR code scan in Expo Go Application on mobile.
Output:
It will help you…
#React Native
✌️ Like this article? Follow me on Twitter and Facebook. You can also subscribe to RSS Feed.
- React Native Image Slider Box Example
- React Native Action Button Example
- React Native Convert Any Input Value in Md5 Example
- React Native CountDown Timer Example
- How to Get IP Address in React Native?
- How to Filter Array of Objects in React Native App?